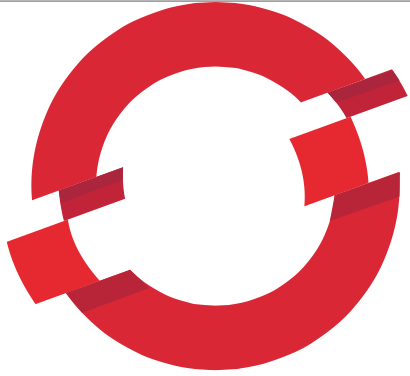
OpenShift is Red Hat’s Platform-as-a-Service (PaaS) that allows developers to quickly develop, host, and scale applications in a cloud environment. With OpenShift you have choice of offerings, including online, on-premise, and open source project options.
With Git, developers can deploy web applications in different languages on the platform. OpenShift makes deployment hassle-free and it is also a free and open source software.
In this tutorial, I’ll make a Rails app with a static page and deploy it to the OpenShift platform. The purpose of this tutorial is to see how the deployment process works.
OpenShift Setup
Create an account for free. A free account gives you 3 small gears with a storage capacity of 1gb per gear. OpenShift Gears are secure containers where applications run within OpenShift Nodes. Nodes are instances of Red Hat Enterprise Linux, which is the foundation of OpenShift. In other words, your application resides on Nodes and runs in secure containers within the nodes called Gears.
Other pricing options exists if you need something different, you can compare plans to know more.
Install rhc
Step one is to install the rhc gem, which contains the OpenShift command line tools:
gem install rhc
This installs the gem rhc and its dependencies. Just like git, rhc has a global configuration that can be completed with:
rhc setup
(Assuming you are installing rhc for the first time, which I believe you are.)
You’ll get a prompt asking you to specify your own Openshift server. For this tutorial, we’ll user the server for Openshift online: openshift.redhat.com. Just hit enter to accept the default.
Next, enter the OpenShift login credentials you used in registering.
OpenShift can create and store a token on disk which allows to you to access the server without using your password. The key is stored in your home directory and should be kept secret. You can delete the key at any time by running 'rhc logout'.
Generate a token now? (yes|no)
If you type yes
, a token will be created and stored on disk. This token allows you to access the server without using your password.
Finally, enter yes
to upload your public SSH key to the OpenShift server to allow key-based SSH access.
NameSpace
The final step to setting this up involves creating a domain. In Openshift, applications are grouped into domains. To create an application, you must first create a domain. Each domain has a unique name, called a namespace.
An OpenShift Online namespace forms part of an application’s URL and is unique to your account. The syntax for an application URL is application–namespace.example.com
. Each username supports a single namespace, but you can create multiple applications within the namespace. If you need multiple namespaces, you need to create multiple accounts using different usernames. Note, you must create a namespace before you can create an application.
OpenShift Online uses a blacklist to restrict the namespaces available to you. The blacklist is maintained on the server. A message warns you that you have chosen a blacklisted name and asks you to choose a different namespace if you try to create or alter a namespace using a blacklisted name. Namespaces may contain a maximum of 16 alphanumeric characters and may not have spaces or symbols.
Enter a unique name after the prompt and you are done. That is all, rhc is now configured.
Generate a Rails App
We need something to deploy. Let’s create a little Rails app to play with on OpenShift. I presume you have the rails
gem installed, if not, gem install rails
will get you going.
Generate the Rails app:
$ rails new tent
$ rails generate controller pages new
This creates a PagesController
with a new
method and view. Edit the new page:
###app/views/new.html.erb
<h1>Welcome to Tent</h1>
<p>This is a test page</p>
Change the route root to:
###config/routes.rb
Rails.application.routes.draw do
root 'pages#new'
end
We want to use Puma as the web server and PostgreSQL for the database. Puma is one of the web servers OpenShift supports. You can choose to use other web servers, such as Unicorn, Thin, or Passenger.
Now go to the Gemfile and add the following:
###Gemfile group :production do gem 'pg' gem 'puma', '2.11.1' end
Run bundle install
after that.
OpenShifting the Rails App
At this point, we want to create a new remote for our app. You need to run this command from the directory above your Rails app (~/parent-directory/rails-app). This is to prevent the creation of a new directory in your app:
rhc app-create tent ruby-2.0 postgresql-9.2
Note: ‘tent’ is the name of the app.
Edit the production configuration in database.yml as shown below:
###config/database.yml
production:
adapter: postgresql
encoding: unicode
pool: 5
database: < %=ENV['OPENSHIFT_APPNAME']%>
host: < %=ENV['$OPENSHIFT_POSTGRESQL_DB_HOST']%>
port: < %=ENV['$OPENSHIFT_POSTGRESQL_DB_PORT']%>
username: < %=ENV['OPENSHIFT_POSTGRESQL_DB_USERNAME']%>
password: < %=ENV['OPENSHIFT_POSTGRESQL_DB_PASSWORD']%>
Preparing to Deploy
Now let’s initialize our local repo:
cd tent
git init
Check the details of your app, you’ll need it for deployment:
rhc show-app tent
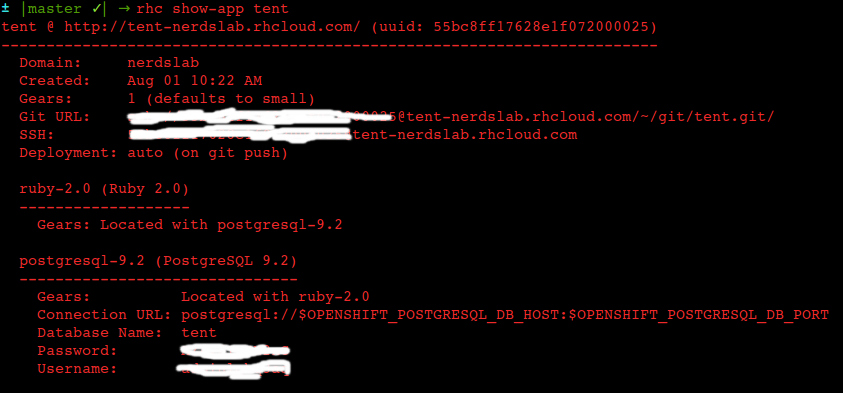
Get the git URL and replace GIT REMOTE URL
with it in the following command:
git remote add openshift GIT REMOTE URL
Let’s merge the remote:
git pull openshift master
You should get a conflict in config.ru, we need to ensure it contains the default content. Open your config.ru and paste in the following:
#config.ru
#This file is used by Rack-based servers to start the application.
require ::File.expand_path('../config/environment', __FILE__)
run Rails.application
Then, commit your changes:
git add .
git commit -m "fixed conflict in config.ru"
I have created an .openshift directory as an example that you can find here. Extract it and override the .openshift directory in your app. The structure of this directory looks like:
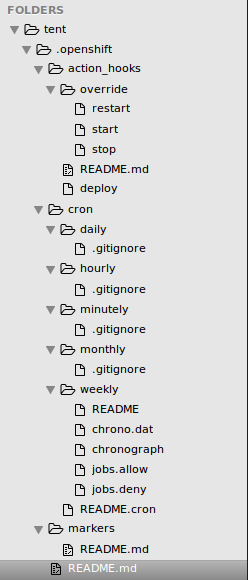
The .openshift directory, located in the home directory of your app is a hidden directory where a user can create action hooks, set markers, and create cron jobs. Action hooks are scripts that are executed directly and can be written in Python, PHP, Ruby, bash, etc.
The example repository above also has a config folder with a puma.rb
file. This file configures Puma and has the following content:
###config/puma.rb
workers Integer(ENV['WEBCONCURRENCY'] || 2)
threadscount = Integer(ENV['MAX_THREADS'] || 5)
threads threads_count, threads_count
preload_app!
rackup DefaultRackup
environment ENV['RACK_ENV'] || 'development'
Copy this file to your local config directory.
Deploy the App
Push the app to the remote server:
git push openshift master
Note: If you get an error while pushing, repeat the pull request and push again.
Now your application is online. Just visit the application URL from your browser, which is http://app-nerdslab.rhcloud.com. For my ‘tent’ app, it’s:
http://tent-nerdslab.rhcloud.com
Migrating the Database
To run a database migration, we need to ssh into our server and set it up:
rhc ssh tent
cd app-root/repo
bundle exec rake db:setup RAILS_ENV=production
If you experience any issues, you can always ssh into your server to fix it using these same commands. Remember that tent in the command above should be replaced with the name of your application.
Checking the Logs
If you ever encounter errors, you’ll want to access the application logs. To check the logs:
rhc ssh tent
cd $OPENSHIFT_LOG_DIR
ls
vim ruby.log #or replace with postgresql.log
Changing the Web Server
The OpenShift ruby cartridge supports, by default, only Passenger running on Apache. But the Advanced Ruby cartridge allows you to use other popular servers, such as Puma, Unicorn, Thin, Rainbows, and, Passenger. In order to change it, we will use a feature of rhc
which allows us to change environment variables. The name of your chosen web server lives in the OPENSHIFT_RUBY_SERVER
environment variable.
To select Puma:
rhc env set OPENSHIFT_RUBY_SERVER=puma -a tent
To take effect, you have to restart the application:
rhc app restart tent
You can check which server is running by using this command:
rhc ssh tent '~/advanced-ruby/bin/control server'
If you are using a Gemfile, ensure you have added the appropriate gem of your selected web server. In this case, it looks like:
###Gemfile
group :production do
gem 'puma', '2.11.1'
end
Conclusion
There are lots of options for hosting a Rails app besides OpenShift. There is a post on SitePoint about Shelly Cloud by Jesse Herrick. Here’s another post on deploying a Rails application to Amazon Web Services (AWS) . Of course, there’s always Heroku. Now, I can recommend OpenShift as a hosting provider for easy deployment.
Frequently Asked Questions (FAQs) about Deploying Rails to OpenShift
What are the benefits of using OpenShift for Ruby on Rails hosting?
OpenShift offers several advantages for Ruby on Rails hosting. It provides a cloud-based platform that allows developers to build, deploy, and scale applications easily. OpenShift supports multiple languages, including Ruby, allowing Rails applications to run seamlessly. It also offers automated build and deployment processes, which can significantly reduce the time and effort required for application deployment. Additionally, OpenShift provides a range of services such as database and messaging services, which can be easily integrated with Rails applications.
How does OpenShift compare to other Ruby on Rails hosting providers?
OpenShift stands out from other hosting providers due to its robust, scalable, and flexible platform. It supports a wide range of programming languages, including Ruby, and offers a variety of services that can be easily integrated with Rails applications. Unlike some hosting providers, OpenShift provides automated build and deployment processes, which can significantly streamline application deployment. Furthermore, OpenShift’s cloud-based platform allows for easy scaling of applications, making it a suitable choice for both small and large Rails applications.
Is OpenShift suitable for beginners in Ruby on Rails?
Yes, OpenShift is suitable for beginners in Ruby on Rails. It provides a user-friendly interface and detailed documentation that can help beginners get started with deploying their Rails applications. Additionally, OpenShift’s automated build and deployment processes can simplify the deployment process for beginners.
How secure is OpenShift for Ruby on Rails hosting?
OpenShift provides a high level of security for Ruby on Rails hosting. It includes built-in security features such as automated security patches and updates, secure container technology, and role-based access control. These features help protect your Rails applications from potential security threats.
Can I scale my Ruby on Rails application on OpenShift?
Yes, you can easily scale your Ruby on Rails application on OpenShift. OpenShift’s cloud-based platform allows you to scale your application up or down based on your needs. This can be particularly useful for handling traffic spikes and ensuring optimal performance of your Rails application.
Does OpenShift support database integration with Ruby on Rails applications?
Yes, OpenShift supports database integration with Ruby on Rails applications. It offers a range of database services, including MySQL, PostgreSQL, and MongoDB, which can be easily integrated with your Rails application.
How reliable is OpenShift for Ruby on Rails hosting?
OpenShift is highly reliable for Ruby on Rails hosting. It provides a robust and stable platform for running Rails applications. Additionally, OpenShift offers high availability and disaster recovery features, ensuring that your Rails application remains accessible and functional at all times.
Can I use OpenShift for free for Ruby on Rails hosting?
OpenShift offers a free tier for developers, which can be used for hosting Ruby on Rails applications. However, the free tier comes with certain limitations, and you may need to upgrade to a paid plan for more resources or advanced features.
How can I migrate my existing Ruby on Rails application to OpenShift?
Migrating your existing Ruby on Rails application to OpenShift involves several steps, including creating a new OpenShift application, setting up your database, and pushing your Rails application code to OpenShift. Detailed instructions for migrating a Rails application to OpenShift can be found in the OpenShift documentation.
What kind of support does OpenShift offer for Ruby on Rails hosting?
OpenShift offers comprehensive support for Ruby on Rails hosting. This includes detailed documentation, a community forum where you can ask questions and share knowledge, and professional support options for more complex issues or requirements.
Kingsley Silas is a web developer from Nigeria. He has a hunger for acquiring new knowledge in every aspect he finds interesting.