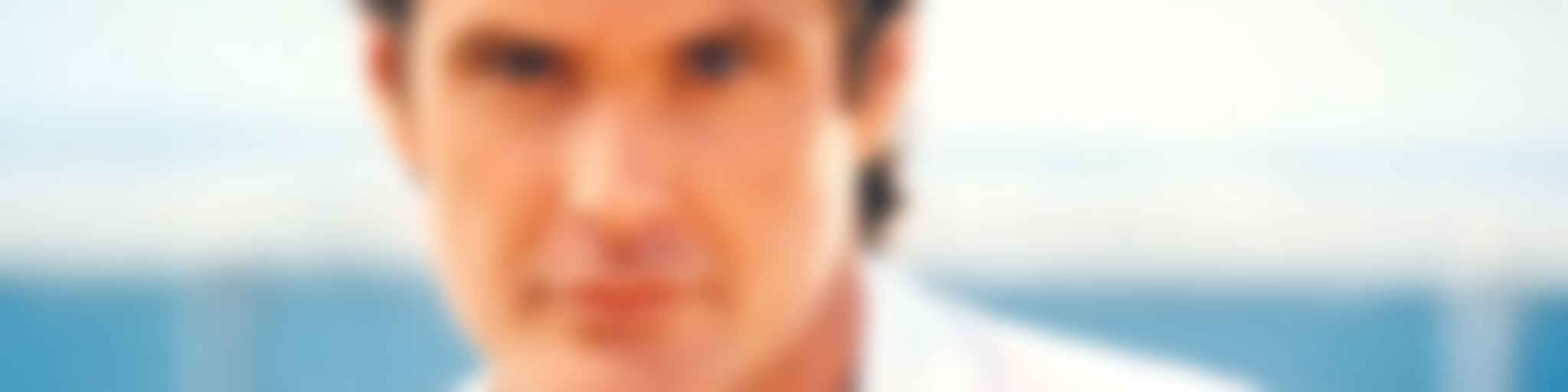
Rescue specific errors. Avoid rescuing StandardError. Don’t rescue Exception.
There are many built-in error classes in Ruby and Rails. Most of these errors are subclasses of Ruby’s StandardError. You can find more information in the relevant Ruby docs.
I'm co-chairing RailsConf 2024 in Detroit May 7–9.
Come and join us
Instead of…
…rescuing Exception
.
def your_method
# do something
rescue Exception => e
# saved ALL THE THINGS
end
Or…
…a non-specific rescue
that implicitly rescues StandardError
.
def your_method
# do something
rescue => e
# saved StandardError and all subclasses
end
Use…
…rescue
on a specific named error.
def your_method
# do something
rescue SpecificProblemError => e
# saved only what you meant to
rescue AnotherProblemError => e
# saved a different kind of error
end
But why?
Ruby’s Exception
is the parent class to all errors. “Great” you might say, “I want to catch all errors”. But you don’t.
Exception
includes the class of errors that can occur outside your application. Things like memory errors, or SignalException::Interrupt
(sent when you manually quit your application by hitting Control-C). These are errors you don’t want to catch in your application as they are generally serious and related to external factors. Rescuing Exception
can cause very unexpected behaviour.
StandardError
is the parent of most Ruby and Rails errors. If you catch StandardError
you’re not introducing the problems of rescuing Exception
, but it is not a great idea. Rescuing all application-level errors might cover up unrelated bugs you don’t know about.
The safest approach is to rescue the error (or errors) you are expecting and deal with the consequences of that error inside the rescue
block.
In the event of an unexpected error in your application you want to know that a new error has occurred and deal with the consequences of that new error inside its own rescue
block.
Being specific with rescue
means your code doesn’t accidentally swallow new errors. You avoid subtle hidden errors that lead to unexpected behaviour for your users and bug hunting for you.
Still running UK’s friendliest, Ruby event on Friday 28th June.
Ice cream + Ruby
Last updated on October 15th, 2017 by @andycroll
An email newsletter, with one Ruby/Rails technique delivered with a ‘why?’ and a ‘how?’ every two weeks. It’s deliberately brief, focussed & opinionated.